Scalar arithmetic operation on Matrix
An Arithmetic operation (addition or subtraction or multiplication or division) is carried out between a matrix and a scalar constant. In which each element of the matrix individually undergoes arithmetic operation by the consultant.
Assume that, A is matrix and c is a scalar constant and an arithmetic operation addition i.e (A + c) The following figure shows how to add scalar constant over a matrix.

The results of a scalar constant addition over a matrix is each elements of the matrix added by the constant c.
Matrix scalar addition
Each elements of the matrix is added by the constant c. The example shown below a 3x3 matrix is added by a scalar constant 2
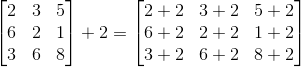
Matrix scalar subtraction
Each elements of the matrix is subtracted by the constant c. The example shown below a 3x3 matrix is subtracted by a scalar constant 4
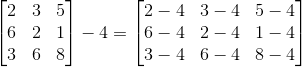
Matrix scalar multiplication
Each elements of the matrix is multiplied by the constant c. The example shown below a 3x3 matrix is multiplied by a scalar constant 2
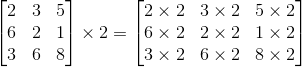
Matrix scalar division
Each elements of the matrix is divided by the constant c. The example shown below a 3x3 matrix is divided by a scalar constant 2
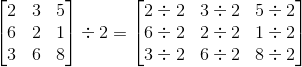
Scalar arithmetic on matrix - Java programming code
The Java program has five static member functions (add, subtract,multiply,divide and power) to carry out arithmetic operation over matrix by a scalar constant.
public class Scalar {
public static Matrix add(Matrix mat,double scalar)
{
Matrix mat2 =new Matrix(mat.getNrow(),mat.getNcol());
for(int r=0;r<mat.getNrow();r++) {
for(int c=0;c<mat.getNcol();c++)
mat2.setElement(r,c, mat.getElement(r,c)+scalar);
}
return mat2;
}
public static Matrix subtract(Matrix mat,double scalar)
{
Matrix mat2 =new Matrix(mat.getNrow(),mat.getNcol());
for(int r=0;r<mat.getNrow();r++) {
for(int c=0;c<mat.getNcol();c++)
mat2.setElement(r,c, mat.getElement(r,c)-scalar);
}
return mat2;
}
public static Matrix multiply(Matrix mat,double scalar)
{
Matrix mat2 =new Matrix(mat.getNrow(),mat.getNcol());
for(int r=0;r<mat.getNrow();r++)
{
for(int c=0;c<mat.getNcol();c++)
mat2.setElement(r,c, mat.getElement(r,c)*scalar);
}
return mat2;
}
public static Matrix power(Matrix mat,double scalar)
{
Matrix mat2 =new Matrix(mat.getNrow(),mat.getNcol());
for(int r=0;r<mat.getNrow();r++)
{
for(int c=0;c<mat.getNcol();c++)
mat2.setElement(r,c, Math.pow(mat.getElement(r,c),scalar));
}
return mat2;
}
public static Matrix divide(Matrix mat,double scalar)
{
Matrix mat2 =new Matrix(mat.getNrow(),mat.getNcol());
for(int r=0;r<mat.getNrow();r++)
{
for(int c=0;c<mat.getNcol();c++)
mat2.setElement(r,c, mat.getElement(r,c)/scalar);
}
return mat2;
}
public static void main(String[] args) {
double vals[][]={{3,1,2},{2,-1,1},{1,3,-1}};
Matrix A =new Matrix(vals);
System.out.println("Matrix A");
System.out.println(A.toString());
System.out.println("Matrix A+5");
Matrix B=Scalar.add(A, 5);
System.out.println(B.toString());
System.out.println("Matrix A-3");
Matrix C=Scalar.multiply(A, 3);
System.out.println(C.toString());
System.out.println("Matrix A.*2");
Matrix D=Scalar.multiply(A, 2);
System.out.println(D.toString());
System.out.println("Matrix A./0.5");
Matrix E=Scalar.divide(A, 0.5);
System.out.println(E.toString());
System.out.println("Matrix A.^2");
Matrix F=Scalar.power(A, 2);
System.out.println(F.toString());
}
}
Scalar arithmetic on matrix - Java programming Output
Matrix A 3.0 1.0 2.0 2.0 -1.0 1.0 1.0 3.0 -1.0 Matrix A+5 8.0 6.0 7.0 7.0 4.0 6.0 6.0 8.0 4.0 Matrix A-3 0.0 -2.0 -1.0 -1.0 -4.0 -2.0 -2.0 0.0 -4.0 Matrix A.*2 6.0 2.0 4.0 4.0 -2.0 2.0 2.0 6.0 -2.0 Matrix A./0.5 6.0 2.0 4.0 4.0 -2.0 2.0 2.0 6.0 -2.0 Matrix A.^2 9.0 1.0 4.0 4.0 1.0 1.0 1.0 9.0 1.0
Comments
Post a Comment