Orthogonal vector
A two or more vectors can be orthogonal to one another in other words, These vectors are perpendicular to each other. To detect whether these two vectors are orthogonal or not, inner-product of these two vectors is used. If the result the inner-product return 0 (zero), these two vectors are orthogonal, otherwise not.
vector X

vector Y

Two vectors X and Y Orthogonal
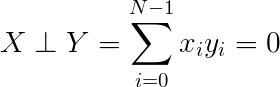
Java code - Orthogonal
The following Java code finds two vectors X and Y are orthogonal or not to each other by inner-product of these two vector. A vector is defined in Java program by a integer array or a double array.
import java.util.Arrays;
public class Vector
{
public static boolean isorthogonal(double vec[],double vec2[])
{
boolean ortho =false;
double inp=0.0;
for(int n=0;n<vec.length;n++)
inp = inp + vec[n] * vec2[n];
if ( inp ==0 )
ortho=true;
return ortho;
}
public static boolean isorthogonal(int vec[],int vec2[])
{
boolean ortho =false;
int inp=0;
for(int n=0;n<vec.length;n++)
inp = inp + vec[n] * vec2[n];
if ( inp ==0 )
ortho=true;
return ortho;
}
public static void main(String[] args)
{
System.out.println( "\nFind Vector V and V2 are orthogonal");
double V[]={5.0 ,1.2 , 2.0};
double V2[]={1.0, 1.2, -2.0};
System.out.println( "\nVector V :" + Arrays.toString(V) );
System.out.println( "Vector V2 :" + Arrays.toString(V2));
boolean status = Vector.isorthogonal(V,V2);
System.out.println( "V and V2 Vector are orthogonal ");
System.out.println( status );
System.out.println( "\nFind Vector U and U2 are orthogonal");
int U[] = {1, 1, -2};
int U2[] = {3, 1, 2};
System.out.println( "\nVector U :" + Arrays.toString(U) );
System.out.println( "Vector U2 :" + Arrays.toString(U2));
boolean status2 = Vector.isorthogonal(U,U2);
System.out.println( "U and U2 Vector are orthogonal ");
System.out.println( status2 );
}
}
Output
Vector V :[5.0, 1.2, 2.0] Vector V2 :[1.0, 1.2, -2.0] V and V2 Vectors are orthogonal false Vector U :[1, 1, -2] Vector U2 :[3, 1, 2] U and U2 Vectors are orthogonal true
Comments
Post a Comment